The power of GitHub Copilot realised
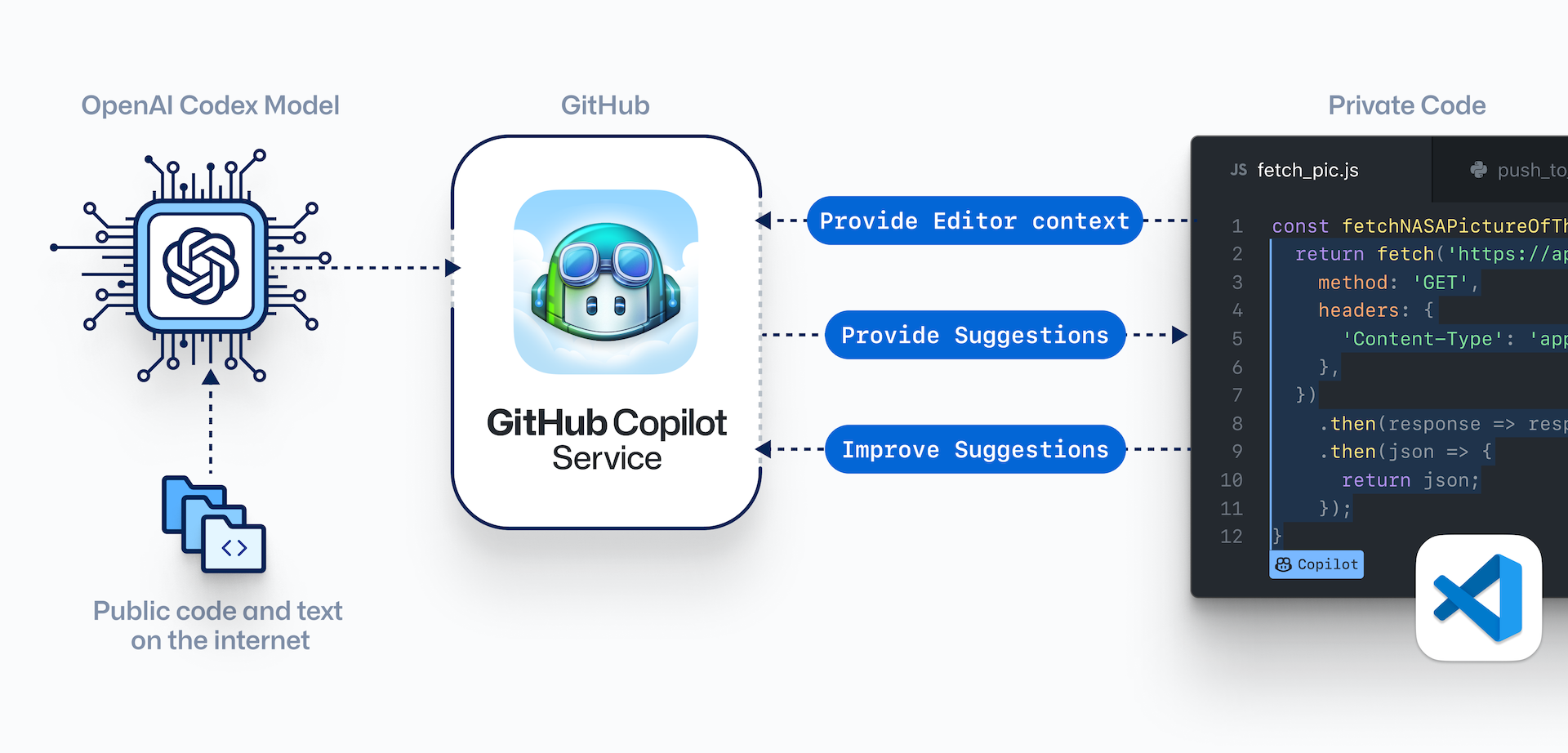
In this article, I explain how GitHub Copilot (GHC) has been valuable to me in my personal, non-work related life.
What is GitHub Copilot?
GitHub Copilot basically autocompletes whole blocks of code for you given some context. For example, when you write a detailed comment just before a function, GHC will autocomplete the whole function for you! GitHub Copilot is powered by the OpenAI Codex. If you are interested to try out GHC, you can sign up to the waitlist on their website. Personally it took 3 days before I was accepted.
What is the OpenAI Codex?
The OpenAI Codex is an AI system that translates natural language to code. That’s it.
How do I set up GitHub Copilot in Visual Studio Code?
All you have to do is search for GitHub Copilot in the extensions market, and sign in to your GitHub account when prompted. Note however that you will only be able to use GitHub Copilot once you have been accepted on the waitlist. For more information, check out their website.
What has GitHub Copilot done for you?
My father who is not too code-savvy had posed to me a little question for his research project: Given a number, how many ways can you split that number into n partitions?
I had trouble understanding the specifics of this question but I broke it down alongside some short discussions with dad to this: Given a target sum m, find all possible ways to make the sum with n terms.
I have provided two examples to help you understand the problem.
Target sum (m): 4
Target number of terms (n): 2
Number of possibilities: 5
[0, 4]
[1, 3]
[2, 2]
[3, 1]
[4, 0]
Target sum (m): 5
Target number of terms (n): 3
Number of possibilities: 21
[0, 0, 5]
[0, 1, 4]
[0, 2, 3]
[0, 3, 2]
[0, 4, 1]
[0, 5, 0]
[1, 0, 4]
[1, 1, 3]
[1, 2, 2]
[1, 3, 1]
[1, 4, 0]
[2, 0, 3]
[2, 1, 2]
[2, 2, 1]
[2, 3, 0]
[3, 0, 2]
[3, 1, 1]
[3, 2, 0]
[4, 0, 1]
[4, 1, 0]
[5, 0, 0]
Now this seemed logical enough to understand so I wrote it down as a comment and created a basic function structure in Python as follows:
''' Given a target sum m, find all possible ways to make the sum with n terms.
'''
def possibilities(m, n):
combos = []
return combos
I hit CTRL + enter
to bring up 10 possible code suggestions and without much thought I picked the first one as it seemed fitting. By this point I hadn’t yet understood the code entirely. I hit Accept Response
and voila, the most elegant piece of code popped out. Mind you this took no more than a minute to do.
When I read the code generated by GHC in detail, it made so much sense. It was both elegant and surprising. I felt as though I had unlocked a new superpower, had hacked life for the first time ever.
''' Given a target sum m, find all possible ways to make the sum with n terms.
'''
def possibilities(m, n):
combos = []
if n == 0:
combos.append([])
elif n == 1:
if m == 0:
combos.append([0])
else:
combos.append([m])
else:
for i in range(m+1):
for c in possibilities(m-i, n-1):
combos.append([i] + c)
return combos
GitHub Copilot writes elegant code
This specific function function proceeds by cases. If there are 0 terms, then the only combination is the empty list. If there is 1 term, then the only combination is the target sum. If there are 2 or more terms, then for every number i
from 0
to m
, repeat finding possibilities which sum to m-i
and n-1
terms. Then join it all up.
You may have noticed that the function could be made slightly better by removing the inner if else clause and producing the following instead.
''' Given a target sum m, find all possible ways to make the sum with n terms.
'''
def possibilities(m, n):
combos = []
if n == 0:
combos.append([])
elif n == 1:
combos.append([m])
else:
for i in range(m+1):
for c in possibilities(m-i, n-1):
combos.append([i] + c)
return combos
So GHC is not perfect but it’s not far from it!
GitHub Copilot saved my time
What would have taken me 2-3 hours to write was complete in a minute! My experience with GitHub Copilot could not be any better. My next objective would be to teach dad how to use GHC for himself, and then he won’t need my help with these types of questions ever again!
Humans need not apply!
If you would like the full Python script, 80% of which has been generated by GHC, you can access it on my GitHub page.